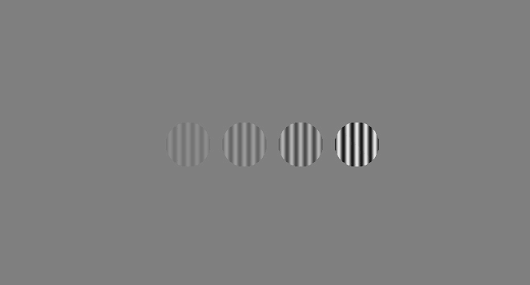
PsychoPy – a basic example
PsychoPy is an excellent tool for preparing psychological experiments in Python.
However, it seems that entry-level examples one can find in the web are rather sophisticated. Hence, I prepared this basic example featuring:
- Installing PsychoPy on Linux
- Displaying a simple stimulus
- Simplest possible keyboard event
Installing PsychoPy on Linux
Open a terminal (5 Ways to Open a Terminal Console Window Using Ubuntu).
Install PsychoPy:
sudo pip install psychopy
I don’t recommend beginners using PsychoPy on Windows. Especially on some older machines. Windows is generally not Python friendly, and installing all dependencies and virtual environments can be harder for a beginner even than installing a dual-boot Linux or just getting some old PC and installing Lubuntu there.
Display simple stimuli
Open some text editor, e.g., Gedit (on Ubuntu), paste and save the following text to a filename named, e.g.: “psychopy_stimulus_example.py”:
# Import modules from psychopy import visual, core # Create a window window = visual.Window([400,400]) # Prepare a fixation point (we will draw it in the next step) fixation = visual.TextStim(window, text='+') # Draw the stimulus fixation.draw() # Refresh/update the window (with the fixation point drawn on) window.flip() # Show the fixation point for 2 seconds core.wait(2.0) # Prepare the first stimulus message = visual.TextStim(window, text='Stimulus!') # Draw the stimulus message.draw() # Show the window after drawing the stimulus window.flip() # Display the stimulus for 2 seconds core.wait(2.0)
Then use the terminal to run the file with a command:
python psychopy_stimulus_example.py
Example based on:
Keyboard example
Now we will run a simple keyboard example script. Copy-paste the following text and save it as “psychopy_keyboard_example.py”:
# Import modules from psychopy import visual, core, event # Create a simple window window = visual.Window([400,400]) # Show the window (it should be empty, but we need it to record keyboard events) window.flip() # An infinite loop while True: # Get keys pressed by the participant keys = event.getKeys() # If the participant strokes "q" if 'q' in keys: # just quit the program core.quit() for key in keys: # Else print the key to the terminal print(key)
Run the example in the terminal with the following command:
python psychopy_stimulus_example.py
This example is also available as a gist: https://gist.github.com/mikbuch/1c5f49b8fa54380b05577e125309221a